Performance Analysis of a Market-Making Strategy in Quantitative Trading (Part 2)
Published:
This article concludes the series and continues from Performance Analysis of a Market-Making Strategy in Quantitative Trading (Part 1).
Step 4: Realised and Unrealised Profit and Loss Calculation
This section evaluates the financial performance of the market-making strategy by calculating both realised and unrealised profit and loss (PnL). The analysis employs the average cost method to dynamically track inventory costs and assess trade outcomes over time. This helps gauge the true profitability of the strategy while keeping tabs on open positions.
Determine Target Inventory
The first step is to infer the target inventory by calculating the median balance held during the trading period. This target acts as a benchmark against which deviations in held inventory are measured. The target inventory serves as a reference point to quantify inventory fluctuations and assess position imbalances.
target_inventory = fills_data['balance'].median()
print("Inferred Target Inventory (median of balance):", target_inventory)
Output:
Inferred Target Inventory (median of balance): 0.5459000000000004
In this example, the median balance indicates a target inventory of approximately 0.546 ETH, suggesting that the strategy aims to maintain its holdings around this level.
Calculate Realised and Unrealised Profit and Loss
Realised PnL is derived from executed trades by comparing the trade price to the average cost of the inventory:
- Buying (
B
) increases inventory and updates the cost basis by adding the purchase price and any associated fees. - Selling (
S
) realises a profit or loss by comparing the sale price with the current average cost of the held inventory. - Cumulative Realised PnL maintains a running total of the profits (or losses) from all completed trades, thus providing a historical perspective on the strategy’s performance.
After each sale, the total inventory and cost basis are adjusted to reflect the new position, ensuring that the average cost remains accurate.
Unrealised PnL, on the other hand, represents the potential profit or loss from the current inventory position based on its deviation from the target inventory:
- When excess inventory is held (i.e. above the target), unrealised PnL assumes that the surplus would be sold at the current bid price.
- When there is a deficit in inventory (i.e. below the target), it assumes that the shortfall would be purchased at the current ask price.
- If there is zero inventory, unrealised PnL is naturally zero since there are no assets to assess.
Realised PnL captures the outcome of completed trades, while unrealised PnL estimates the market value of open positions and highlights potential risks if the held inventory deviates significantly from the target.
The following code iterates over each trade in the fills data to:
- Update the cost basis and inventory based on whether the trade was a buy or a sell.
- Calculate realised profit or loss on sales.
- Adjust the cumulative realised PnL.
- Compute the current average cost of the remaining inventory.
- Estimate unrealised PnL based on deviations from the target inventory.
# Compute realized PnL using an average cost method
# At each fill, update cost basis and inventory
total_inventory = 0.0
total_cost = 0.0
realized_pnl_list = []
avg_cost_list = []
unrealized_pnl_list = []
cumulative_realized_list = []
cumulative_realized = 0.0
for row in fills_data.itertuples():
if row.side == 'B':
# If buying, increase inventory and update total cost (including fees)
total_cost += (row.fill_prc * row.fill_qty) + row.fee_usd
total_inventory += row.fill_qty
realized = 0.0
elif row.side == 'S':
# If selling, realize PnL using the average cost of the current inventory
if total_inventory <= 0:
realized = 0.0
else:
avg_cost = total_cost / total_inventory # Calculate the average cost of held inventory
qty_sold = min(row.fill_qty, total_inventory) # Binance doesn't sell more than it has
cost_of_sale = avg_cost * qty_sold # Cost basis for the quantity sold
sale_proceeds = (row.fill_prc * qty_sold) - row.fee_usd # Cash received from the sale minus fees
realized = sale_proceeds - cost_of_sale # Profit or loss on the trade
# Reduce total inventory and adjust cost basis accordingly
total_cost -= cost_of_sale
total_inventory -= qty_sold
# Update cumulative realized PnL
cumulative_realized += realized
realized_pnl_list.append(realized)
cumulative_realized_list.append(cumulative_realized)
# Compute the current average cost of the remaining inventory
current_avg_cost = total_cost / total_inventory if total_inventory > 0 else 0.0
avg_cost_list.append(current_avg_cost)
# Estimate unrealized PnL based on the deviation from the target inventory
if total_inventory == 0:
unrealized = 0.0
# If holding excess inventory, assume it would be sold at the current bid price
elif total_inventory > target_inventory:
deviation = total_inventory - target_inventory
unrealized = deviation * (row.bid_prc - current_avg_cost)
# If holding less than target inventory, assume buying the shortfall at the current ask price
elif total_inventory < target_inventory:
deviation = target_inventory - total_inventory
unrealized = -deviation * (current_avg_cost - row.ask_prc)
else:
unrealized = 0.0
unrealized_pnl_list.append(unrealized)
Store Computed PnL Metrics
After processing all trades, the computed metrics are appended to the dataset. These metrics include:
- Realised PnL - Profit or loss from executed trades.
- Cumulative Realised PnL - The running total of realised gains or losses.
- Average Cost of Open Inventory - The current cost basis of the remaining holdings.
- Unrealised PnL - The estimated profit or loss from the remaining inventory.
- Total PnL - The sum of realised and unrealised PnL, reflecting the overall profitability of the strategy.
# Append computed metrics to fills_data
fills_data['realized_pnl'] = realized_pnl_list
fills_data['cumulative_realized'] = cumulative_realized_list
fills_data['avg_cost_open'] = avg_cost_list
fills_data['unrealized_pnl'] = unrealized_pnl_list
fills_data['total_pnl'] = fills_data['cumulative_realized'] + fills_data['unrealized_pnl']
Evaluate Final Performance
The final performance metrics are then displayed.
# Overall performance metrics
print(f"Final Realized PnL: ${fills_data['cumulative_realized'].iloc[-1]:.2f}")
print(f"Final Unrealized PnL: ${fills_data['unrealized_pnl'].iloc[-1]:.2f}")
print(f"Total PnL: ${fills_data['total_pnl'].iloc[-1]:.2f}")
print(f"Total Fees Paid: ${fills_data['fee_usd'].sum():.2f}")
Output:
Final Realized PnL: $20.29
Final Unrealized PnL: $0.06
Total PnL: $20.35
Total Fees Paid: $5.41
Final Realised PnL is $20.29, representing the profit secured from fully executed, closed trades. Final Unrealised PnL is $0.06, indicating that the profit or loss from open positions is negligible. Total PnL is $20.35, the sum of realised and unrealised profit, while Total Fees Paid is $5.41, reflecting the trading costs incurred and deducted from the gross profits.
Overall, the strategy is profitable with controlled inventory levels, as realised profit significantly exceeds both unrealised PnL and fee costs. After accounting for fees, the strategy delivers a modest profit with low exposure to unrealised market risk. The stable target inventory and close alignment between realised profits and inventory adjustments suggest effective inventory management.
Step 5: Profit and Loss Breakdown
This section breaks down the realised profit and loss (PnL) by different trade dimensions to better understand the contributions from various aspects of the strategy.
Break Down Realised PnL by Trade Side
Realised PnL is aggregated by trade side to reveal the performance of buy and sell transactions. This breakdown helps distinguish the profitability of each side of the market-making operation.
# Realized PnL by trade side
realized_pnl_by_side = fills_data.groupby('side')['realized_pnl'].sum()
print("Realized PnL by Trade Side:")
for side, pnl in realized_pnl_by_side.items():
trade_type = "Buy" if side == "B" else "Sell"
print(f" - {trade_type}: ${pnl:.2f}")
Output:
Realized PnL by Trade Side:
- Buy: $0.00
- Sell: $20.29
The output indicates that there is no realised profit from buy trades, while sell trades contribute a realised profit of $20.29. This makes sense because profit is only realised through selling.
Assess Realised PnL by Liquidity Role
Next, the realised PnL is grouped by liquidity role (Maker/Taker) to assess which role contributes more to profitability. This analysis can offer insights into how different execution types affect the overall performance.
# Realized PnL by liquidity role
realized_pnl_by_liquidity = fills_data.groupby('liquidity')['realized_pnl'].sum()
print("Realized PnL by Liquidity Role:")
for role, pnl in realized_pnl_by_liquidity.items():
print(f" - {role}: ${pnl:.2f}")
Output:
Realized PnL by Liquidity Role:
- Maker: $16.33
- Taker: $3.96
Here, the majority of realised profit comes from acting as a Maker (16.33 dollars), while Taker trades contribute a smaller amount (3.96 dollars). This suggests that the strategy is prioritising passive order placement, as maker trades benefit from capturing the bid-ask spread along with lower fees or exchange rebates. Conversely, the lower realised profit from Taker orders indicates that aggressive execution is used sparingly, likely as a risk management measure to quickly adjust inventory when market conditions become unfavourable.
Step 6: Time-Series Analysis of Profit and Loss
This section examines how the strategy’s total PnL evolves over time by analysing its daily fluctuations. Tracking PnL on a time-series basis helps identify trends, volatility, and potential inefficiencies in the market-making approach.
Visualise Daily Total PnL
A time-series plot of daily total PnL is generated to observe how profitability changes over time. Each day’s PnL is calculated as the difference between the total PnL at the end of consecutive days.
# Daily total PnL time series plot
fills_data['date'] = fills_data.index.date
daily_pnl = fills_data.groupby('date')['total_pnl'].last().diff().fillna(0)
plt.figure(figsize=(12,6))
plt.plot(daily_pnl.index.astype(str), daily_pnl.values, marker='o', linestyle='-')
plt.xlabel('Date')
plt.ylabel('Daily Total PnL (USD)')
plt.title('Daily Total PnL Time Series')
plt.xticks(rotation=45)
plt.tight_layout()
plt.show()
Output:
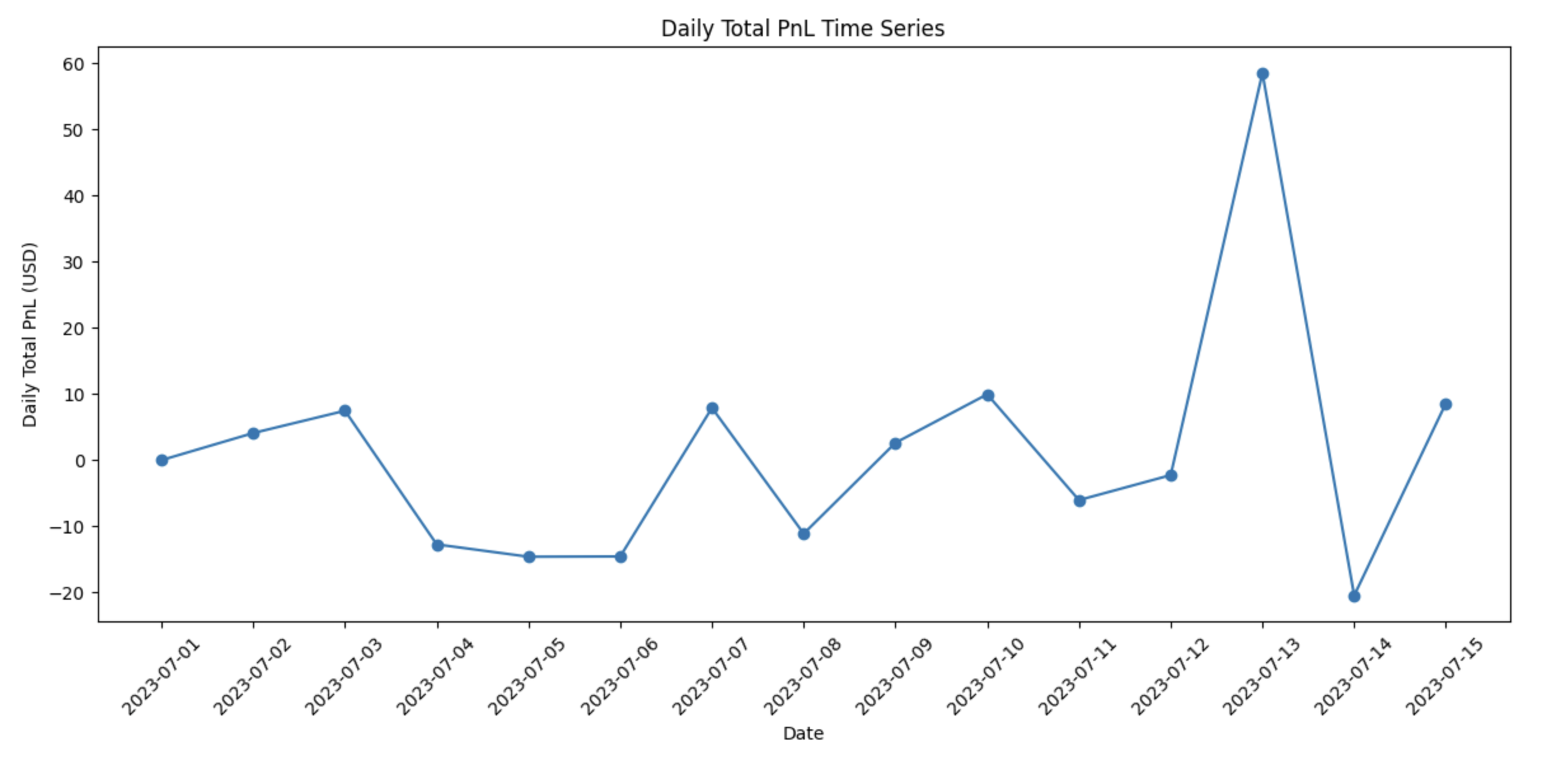
The strategy’s PnL varies from day to day. This indicates sensitivity to market conditions and price movements. Despite this volatility, multiple days show net gains, indicating that the market-making approach remains profitable over the long term. However, significant drawdowns may suggest inefficiencies in inventory management or increased reliance on taker orders at unfavourable prices.
Step 7: Price Behaviour Analysis After Fills
This section examines the immediate market reaction following each fill by analysing the 1-minute return. Focusing on this short interval isolates the direct effect of a trade, reducing interference from later market fluctuations, and provides a robust measure of execution quality and potential slippage. Other time windows (e.g. 5 or 15 minutes) might blend these immediate effects with broader market movements or news events that are less directly tied to the specific trade.
Merge Future Market Data
For each fill, the timestamp is advanced by one minute to capture the corresponding market prices (bid and ask) at that future moment. This technique ensures that the subsequent market reaction is accurately recorded, reflecting any rapid price adjustments post-execution.
# Convert both indexes to nanosecond precision
market_data.index = market_data.index.astype('datetime64[ns]')
fills_data.index = fills_data.index.astype('datetime64[ns]')
# Create a new column for timestamp shifted by one minute.
fills_data['timestamp_plus_1min'] = fills_data.index + pd.Timedelta(minutes=1)
# Merge forward bid/ask prices from market_data
fills_data = pd.merge_asof(
fills_data,
market_data[['bid_prc', 'ask_prc']].rename(columns={'bid_prc': 'bid_1min', 'ask_prc': 'ask_1min'}),
left_on='timestamp_plus_1min',
right_index=True,
direction='forward'
)
Calculate 1-Minute Returns by Trade Side
The 1-minute return is calculated separately for buy and sell fills. For buy fills, the return is derived from the difference between the future ask price and the fill price; for sell fills, it is based on the difference between the fill price and the future bid price. This calculation quantifies the immediate profitability of each trade and identifies any short-term execution advantages.
# Compute 1-minute return based on trade side
fills_data['return_1min'] = np.where(
fills_data['side'] == 'B',
(fills_data['ask_1min'] - fills_data['fill_prc']) / fills_data['fill_prc'],
(fills_data['fill_prc'] - fills_data['bid_1min']) / fills_data['fill_prc']
)
Visualise 1-Minute Return Distribution
A histogram is used to depict the distribution of 1-minute returns for both trade sides. This visualisation highlights the frequency and range of positive and negative returns. For example, a slightly higher average return for buy fills may indicate a marginally more favourable market response when entering long positions. Such analysis aids in assessing execution performance and refining trade timing.
# Plot the distribution of 1-minute returns by trade side
plt.figure(figsize=(10,6))
sns.histplot(data=fills_data, x='return_1min', hue='side', kde=True, bins=30)
plt.title("Distribution of 1-Minute Returns After Fills")
plt.xlabel("1-Minute Return")
plt.ylabel("Frequency")
plt.show()
Output:
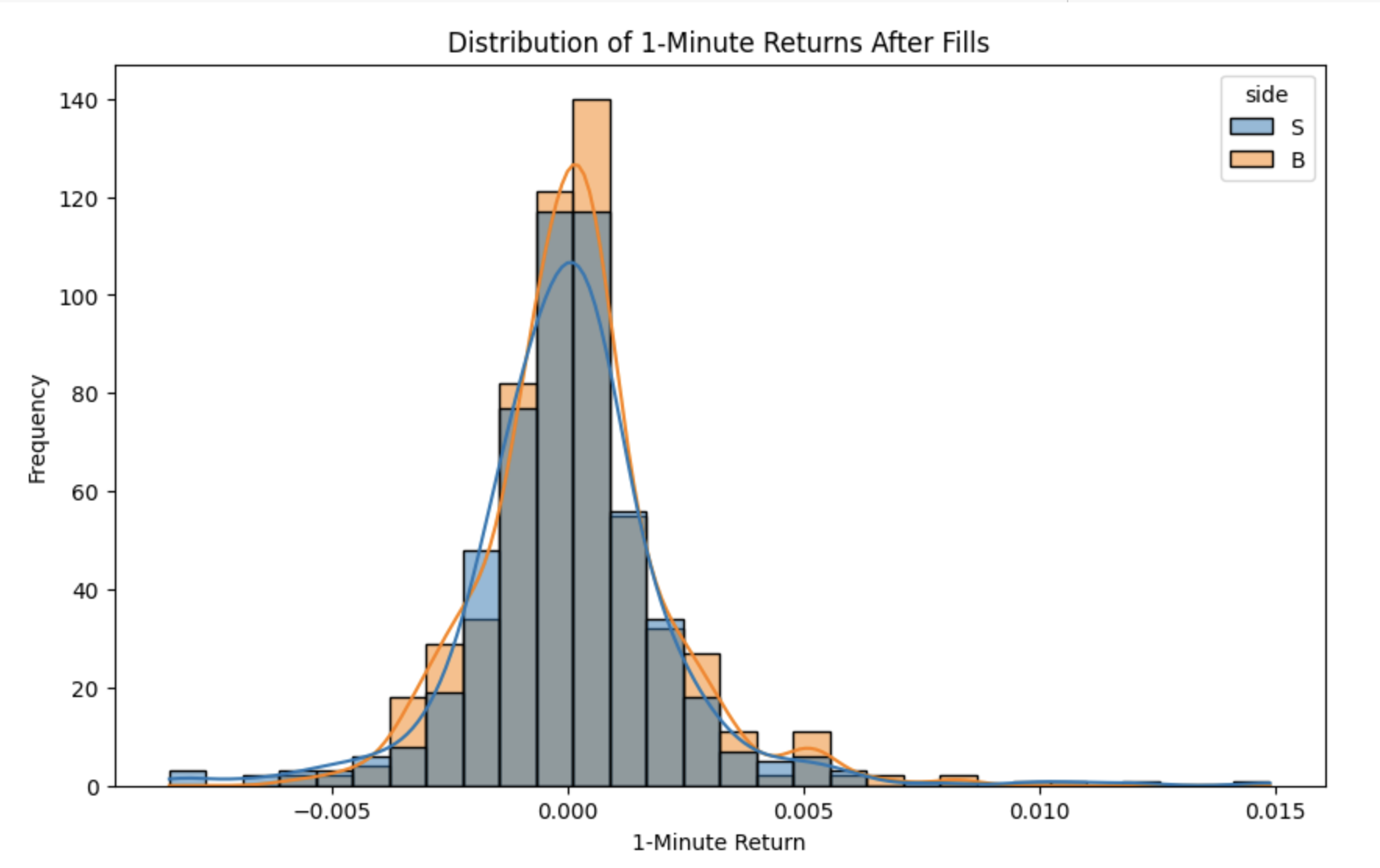
The 1-minute returns for both buy (B) and sell (S) fills cluster around zero. It means prices typically do not move dramatically in the minute after a fill. The bell-shaped curves imply that small price movements (positive/negative) are more common than large swings, indicating relatively efficient market conditions. This pattern implies that market participants quickly absorb new orders without causing significant short-term dislocation, which can be advantageous for strategies aiming to capture small, consistent profits through frequent trades.
Examine 1-Minute Returns by Trade Side
Presenting average returns separately for each side clarifies differences in short-term performance between buy and sell orders. This practice reveals whether market conditions around execution systematically favour one action over the other. A comparison of mean 1-minute returns highlights which side tends to achieve a slightly better outcome.
# Summary statistics for 1-minute returns by trade side
print("Average 1-Minute Return by Side:")
summary_stats = fills_data.groupby('side')['return_1min'].mean().reset_index()
display(summary_stats)
Output:
Average 1-Minute Return by Side:
side return_1min
0 B 0.000122
1 S 0.000028
Buy fills show a mean return of 0.000122, while sell fills stand at 0.000028, indicating a marginal edge for buying in the immediate timeframe. This result may reflect a mild upward bias or faster price recovery after purchase. A higher average return for buy trades could justify stronger inventory replenishment if the market frequently rebounds soon after entry. It may also lead to a review of quoting widths on the sell side to reduce potential slippage or secure gains more efficiently.
Assess Performance Metrics
A broader set of measures, such as win rate, average gain, and average loss, demonstrates how often trades turn a profit within the one-minute window and the typical magnitude of those gains or losses. The win rate captures the proportion of trades that realise a positive return at the one-minute mark, while average gain and loss detail how much is typically won or lost during this brief period.
# Performance Metrics
win_rate = (fills_data['return_1min'] > 0).mean() * 100
avg_gain = fills_data[fills_data['return_1min'] > 0]['return_1min'].mean()
avg_loss = fills_data[fills_data['return_1min'] < 0]['return_1min'].mean()
print(f"Win Rate: {win_rate:.2f}%")
print(f"Average Gain: {avg_gain:.6f}")
print(f"Average Loss: {avg_loss:.6f}")
Output:
Win Rate: 51.29%
Average Gain: 0.001414
Average Loss: -0.001360
A win rate above 50% means the price moves on average in the strategy’s favor just over half the time within the first minute after a fill. It suggests that small yet consistent gains may accumulate over multiple trades. Even a modest advantage in these figures can have a material influence on long-term returns. This highlights the importance of careful execution timing and continuous monitoring of fills in a market-making strategy.
Step 8: Trade Execution and Liquidity Metrics
This section evaluates the strategy’s trade execution and liquidity by summarising key metrics along two dimensions: trade side (Buy/Sell) and liquidity role (Maker/Taker). These summaries reveal how different order types contribute to overall performance and assist in assessing execution efficiency.
Break Down Trade Activity
Trades are classified as either buy or sell, and for each category, metrics such as the total number of trades, average fill quantity, total traded volume, and average fill price are computed. This breakdown illustrates the balance between buying and selling activities, which is crucial for maintaining effective inventory management.
# Create a summary of trade statistics based on the 'side' column (Buy/Sell)
trade_summary = fills_data.groupby('side').agg(
trades=('order_id', 'count'),
avg_fill_qty=('fill_qty', 'mean'),
total_volume=('fill_qty', 'sum'),
avg_fill_price=('fill_prc', 'mean')
).reset_index()
print("Trade Summary by Side:")
display(trade_summary)
Output:
Trade Summary by Side:
side trades avg_fill_qty total_volume avg_fill_price
0 B 580 0.067994 39.4367 1934.553155
1 S 543 0.072369 39.2963 1934.720424
The near parity between 580 buy trades (averaging ~0.068 ETH each, totalling ~39.44 ETH) and 543 sell trades (averaging ~0.072 ETH each, totalling ~39.30 ETH) suggests tight execution and effective inventory management.
Assess Liquidity Contribution
Trades are also grouped by liquidity role to evaluate the impact of different order types on profitability. Liquidity refers to how easily an asset can be bought or sold in the market without significantly affecting its price. Maker orders provide liquidity and often earn lower fees or exchange rebates, while taker orders are executed immediately against existing orders and typically incur higher costs. These roles also reflect different execution strategies: maker orders are considered passive since they add liquidity to the market, whereas taker orders are viewed as aggressive because they remove liquidity.
# Create a summary of liquidity statistics grouping by the 'liquidity' column (Maker/Taker)
liquidity_summary = fills_data.groupby('liquidity').agg(
trades=('order_id', 'count'),
avg_fill_qty=('fill_qty', 'mean'),
total_volume=('fill_qty', 'sum'),
avg_fill_price=('fill_prc', 'mean'),
total_realized_pnl=('realized_pnl', 'sum')
).reset_index()
print("\nLiquidity Summary:")
display(liquidity_summary)
Output:
Liquidity Summary:
liquidity trades avg_fill_qty total_volume avg_fill_price total_realized_pnl
0 Maker 1038 0.063687 66.1076 1935.721599 16.328486
1 Taker 85 0.148534 12.6254 1921.352941 3.960174
The liquidity summary reveals that maker orders dominate, contributing the majority of the realised profit (16.33 dollars), while taker orders, although less frequent and larger in size, contribute a smaller profit (3.96 dollars). This indicates that the strategy predominantly employs passive order placement to capture the bid-ask spread and benefit from lower fees or exchange rebates, with taker orders used sparingly for quick inventory adjustments when market conditions deteriorate.
Closing Remarks
The strategy achieved consistent profitability over the 15-day period by leveraging passive (maker) orders to minimise costs and capture the bid-ask spread efficiently. Maintaining inventory near a target level helped manage risk and confirmed the intended dynamic adjustment between cash flow and inventory.
However, a 15-day sample is too short to capture the full range of market conditions. Extending the analysis across various liquidity regimes and volatility periods is necessary to assess long-term resilience. Also, the reliance on maker orders exposes the strategy to adverse selection risks, which could be mitigated by refining quoting parameters and incorporating real-time market signals.
While the strategy shows promise, further validation under diverse market environments is essential to confirm its scalability and risk-adjusted performance.